WP REST APIを使って投稿を出したかったのですが、REST APIで呼び出せる情報が多すぎてJSONが少し重たかったので、自分に必要なものだけを出力しているJSONファイルを作ることにしました。
(エンドポイントを作る、というようです)
目次
通常投稿の独自エンドポイントを作る
- タイトル
- URL(パーマリンク)
- 抜粋
- 投稿者
- アイキャッチ画像のサムネイル
今回はこの投稿を5件まで出すJSONを作ります。
出し方
functions.phpに下記のようにエンドポイントを使いする記述を書きます。
function add_rest_original_endpoint(){
register_rest_route( 'wp/custom', '/posts', array(
'methods' => 'GET',
'callback' => 'get_posts_original',
));
}
add_action('rest_api_init', 'add_rest_original_endpoint');
function get_posts_original() {
$contents = array();//return用の配列を準備
$myQuery = new WP_Query();//取得したいデータを設定
$param = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$myQuery->query($param);
if($myQuery->have_posts()):
while ( $myQuery->have_posts() ) : $myQuery->the_post();
$title = get_the_title();
$linkurl = get_the_permalink();
$excerpt = get_the_excerpt();
$author = get_the_author();
$thumb = get_the_post_thumbnail_url(0,'full');
array_push($contents, array(
"title" => $title,
"linkurl" => $linkurl,
"excerpt" => $excerpt,
"author" => $author,
"thumb" => $thumb
));
endwhile;
endif;
return $contents;
}
'wp/custom', '/posts'
の部分によって、 [WordPressのURL]/wp-json/wp/custom/postsというJSONが出せます。
JSONの中身はこんな感じ。
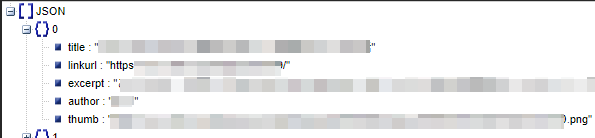
※JSONの構造はブラウザで見てもよく分からないので、 http://jsonviewer.stack.hu/ の力を借りて内容を確認しました。
ページに表示させる(jQuery)
出力したAPIを使って、jQueryでページ上に投稿記事の一覧を表示します。
(function($, root, undefined) {
$.ajax({
type: 'GET',
url: '[★WordPressのURL★]/wp-json/wp/custom/posts',
dataType: 'json'
}).done(function(json){
var html = '';
$.each(json, function( i, row ) {
var title = row.title;
var link = row.linkurl;
var author = row.author;
var excerpt = row.excerpt;
var thumbnail
if( row.thumb ) {
thumbnail = row.thumb;
}else{
thumbnail ='[アイキャッチ画像が無いときの代わりのサムネイル]';
}
html += '<article>';
html += '<a href="' + link + '">';
html += '<div class="eyecatch"><img src="' + thumbnail + '"></div>';
html += '<h3>' + title + '</h3>';
html += '<p class="author">' + author + '</p>';
html += '<div class="content">' + excerpt + '</div>';
html += '</a>';
html += '</article>';
});
$('#wordpress_blog').append(html)
}).fail(function(json){
console.error('WordPress JSON error')
});
})(jQuery, this);
これで、ページ上に無事に表示されました!
参考
神戸のWebデザイナー イラストスイ...
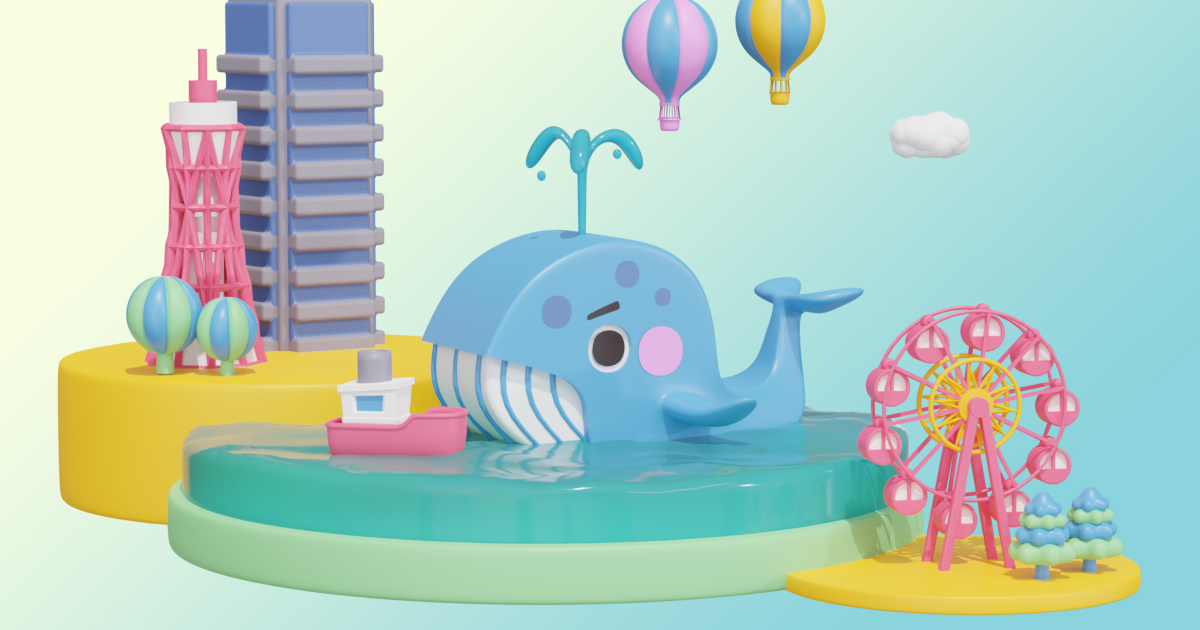
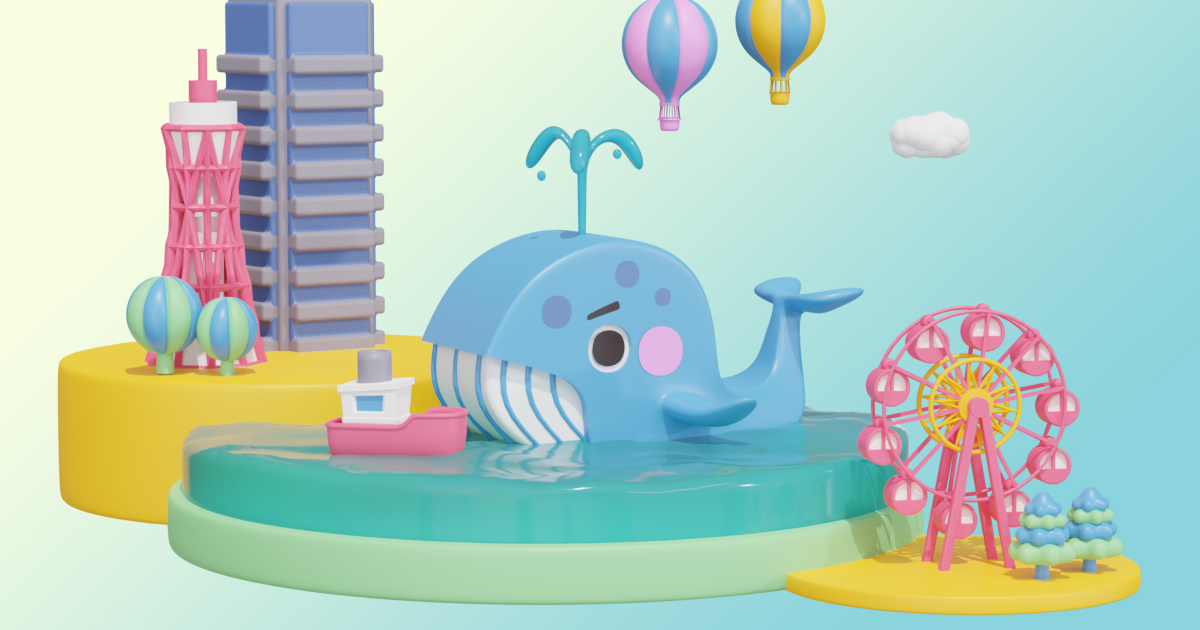
404: ページが見つかりませんでした | 神戸のWebデザイナー イラストスイッチ
神戸市にてWEB制作を行っています。WordPressの導入やイラスト作成お任せください。
https://knowledge.cpi.ad.jp/cms/wp-rest-api2/
カスタム投稿タイプのエンドポイントをつくる
さて、今度は通常投稿ではなく、カスタム投稿タイプです。
- カスタム投稿タイプの設定で、REST APIをtrueにする必要がある
- カスタムフィールドの内容を表示したいときはfunctions.phpに追記しないといけない
という部分以外は、大体一緒です。
function wp_rest_api_alter() {
//Advanced Custom Fieldに対応させるコード
$params = array(
'get_callback' => function($data, $field, $request, $type){
if (function_exists('get_fields')) {
return get_fields($data['id']);
}
return [];
},
'update_callback' => null,
'schema' => null,
);
register_rest_field( 'page', 'fields', $params );
register_rest_field( 'post', 'fields', $params );
}
add_action( 'rest_api_init', 'wp_rest_api_alter');
add_action('rest_api_init', function() {
//REST APIのURLを作る
register_rest_route( 'wp/v2', '/custom_rest_api', array(
'methods' => 'GET',
'callback' => 'custom_rest_api',
));
});
function custom_rest_api( ) {
//REST API の中身
$contents = array();
$myQuery = new WP_Query();
$param = array(
'post_type' => 'product',
'posts_per_page' => -1,
);
$myQuery->query($param);
if($myQuery->have_posts()):
while ( $myQuery->have_posts() ) : $myQuery->the_post();
$ID = get_the_ID();
$title = get_the_title();
$custom = get_field('product_custom_field');
array_push($contents, array(
"title" => $title,
"id" => $ID,
"custom" => $custom
));
endwhile;
endif;
return $contents;
}
参考
https://qiita.com/hisahayashi/items/1617bd0bd494da2a9049
https://qiita.com/RinT_T/items/808941ba52ffa4b51833
コメント